Mastering Real-Time Data Integration: Leveraging Pyth Oracle for Decentralized Applications
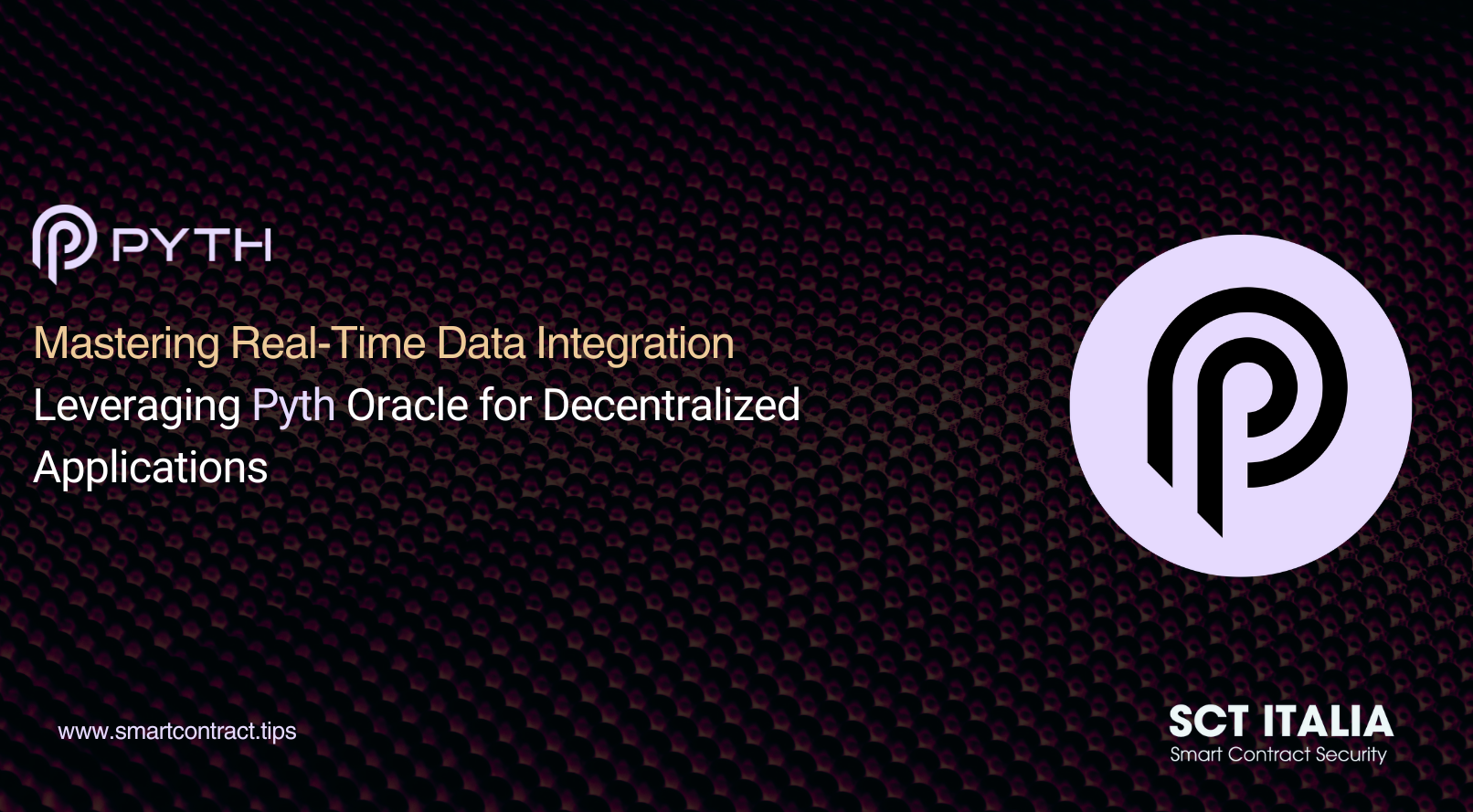
Introduction
The world of blockchain and decentralized finance (DeFi) relies heavily on accurate, real-time data to enable smart contracts to interact seamlessly with off-chain information. Oracles play a pivotal role in bridging the gap between on-chain and off-chain data. Among the innovative oracle solutions, Pyth Network has emerged as a robust platform designed to deliver high-frequency, low-latency price feeds for DeFi and other decentralized applications.
Pyth Network distinguishes itself through its direct connection with institutional-grade data providers, ensuring reliable and precise price data. Whether you are building a lending protocol, decentralized exchange (DEX), or any other smart contract that requires up-to-date price information, Pyth offers a compelling solution.
In this article, we will:
- Explain what Pyth Oracle is and why it is important.
- Provide a detailed walkthrough of integrating Pyth Oracle into Solidity smart contracts.
- Discuss best practices for using Pyth in your projects.
- Demonstrate practical examples with code to fetch and utilize price data.
- Address potential security risks and mitigation strategies when using Pyth Oracle.
By the end of this guide, you will have a comprehensive understanding of how to leverage Pyth Oracle for building reliable and data-driven smart contracts.
What is Pyth Network?
Pyth Network is a decentralized oracle network (DON) focused on delivering real-time financial data such as cryptocurrency prices, equity values, forex rates, and commodities data. It sources its information directly from institutional providers like major trading firms, exchanges, and market makers.
Pyth operates differently from traditional oracles. Instead of continuously pushing data on-chain (which can be expensive), it uses a pull-based model. The data is broadcast off-chain and can be requested on-chain when needed, saving gas fees and reducing latency.
Key Features of Pyth Network:
- High-Frequency Updates: Price feeds are updated every few seconds to reflect real-time market movements.
- Low Latency: Designed to minimize delays, ensuring that price data remains fresh and reliable.
- Cross-Chain Support: Pyth supports multiple blockchain ecosystems, including Solana, Ethereum, Aptos, and more.
- Cost-Efficient: Prices are delivered on-demand, reducing unnecessary on-chain data updates and associated gas costs.
- Institutional Quality: Data is sourced from trusted, high-volume providers in the financial sector.
How Pyth Oracle Works
Pyth operates using a two-step process:
- Off-Chain Data Aggregation: Data providers supply price information to Pyth, which aggregates and broadcasts it to the network. Each price feed is identified by a unique price ID.
- On-Chain Price Pull: Smart contracts can request specific price data by providing the corresponding
priceId
. The price data is delivered with an attestation that cryptographically verifies its authenticity.
The ability to fetch price data on-demand ensures that you only pay for what you use, making it ideal for applications where cost efficiency is critical.
Integrating Pyth Oracle into Solidity Contracts
Below is a step-by-step guide to integrating Pyth Network into your Solidity smart contracts.
Step 1: Import the Pyth Interface
To interact with the Pyth Network, you need to import the Pyth contract interface.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
interface IPyth {
function getPrice(bytes32 priceId) external view returns (int64 price, uint64 publishTime);
}
priceId
: The unique identifier for the specific price feed you need.getPrice
: A function that returns the latest price and its associated timestamp.
Step 2: Set Up Your Contract
Create a smart contract and initialize the Pyth oracle contract address.
contract PythOracleExample {
IPyth public pyth;
// Initialize the contract with the Pyth oracle address
constructor(address _pythAddress) {
pyth = IPyth(_pythAddress);
}
}
Replace _pythAddress
with the deployed address of Pyth’s smart contract on your target network (e.g., Ethereum).
Step 3: Fetching Price Data
Add a function to fetch the latest price for a given price feed ID.
function getPriceData(bytes32 priceId) public view returns (int64 price, uint64 timestamp) {
// Fetch price data from the Pyth oracle
(price, timestamp) = pyth.getPrice(priceId);
return (price, timestamp);
}
Here, priceId
represents the specific asset you want to retrieve, such as BTC/USD.
Practical Example: Fetch BTC/USD Price
To demonstrate, let’s fetch the BTC/USD price. Assume that the price feed ID for BTC/USD is 0xe62df6df
(replace it with the actual ID for your network you can find them here : https://www.pyth.network/developers/price-feed-ids).
function getBTCPrice() public view returns (int64 price) {
// Replace this with the correct BTC/USD price ID
bytes32 btcPriceId = 0xe62df6df;
(price, ) = getPriceData(btcPriceId);
return price;
}
When you deploy and interact with the contract, calling getBTCPrice
will return the latest BTC/USD price.
Expected Output
For example:
BTC/USD Price: 100,500 * 1e6 (scaled value)
Timestamp: 1734620766
The price might be scaled, so you must handle decimal adjustments in your application.
Security Risks and Mitigation Strategies
While Pyth Network is designed to be reliable and secure, there are some risks associated with using oracles. Understanding and mitigating these risks is crucial to building secure smart contracts.
1. Stale Price Data
If a price feed is not updated frequently, your smart contract might rely on outdated information, leading to incorrect calculations or decisions.
Mitigation:
- Use
getPriceNoOlderThan
to ensure that fetched price data is recent.
function getPriceNoOlderThan(bytes32 priceId, uint64 maxAge) public view returns (int64 price, uint64 timestamp) {
(price, timestamp) = pyth.getPrice(priceId);
require(block.timestamp - timestamp <= maxAge, "Price data is stale");
return (price, timestamp);
}
Here, maxAge
is the maximum allowable age for the price data in seconds.
2. Data Integrity Attacks
Price data could be manipulated if the attestation mechanism is compromised.
Mitigation:
- Validate data authenticity by checking Pyth’s cryptographic signatures.
- Use trusted libraries or pre-verified contracts for integration.
3. Price Feed Downtime
If a price feed becomes unavailable, it could disrupt the functionality of your smart contract.
Mitigation:
- Implement fallback mechanisms to handle downtime gracefully.
- Use multiple oracle solutions as redundancy.
4. Over-Reliance on Single Oracle Provider
Relying solely on one oracle provider increases centralization risks.
Mitigation:
- Combine data from multiple oracle networks for better decentralization and accuracy.
Best Practices for Using Pyth Oracle
- Validate Data Freshness: Always check the
publishTime
to ensure the fetched price is up-to-date. - Optimize Gas Usage: Pull price data only when required to avoid unnecessary gas costs.
- Handle Scaling: Pyth prices may be scaled (e.g., multiplied by 1e6). Ensure your smart contract or front-end handles the decimals correctly.
- Secure Contract Interactions: Use access controls to prevent unauthorized entities from calling critical functions.
- Monitor Oracle Performance: Regularly check the availability and reliability of Pyth feeds.
Advanced Use Cases for Pyth Oracle
Pyth Oracle’s real-time and institutional-grade data unlocks a range of advanced applications across various domains:
1. Dynamic Interest Rate Protocols
Lending platforms can leverage Pyth Oracle to implement dynamic interest rates based on real-time market conditions. For instance:
- Borrowing rates can adjust dynamically based on the volatility of collateral assets.
- Interest rates can be pegged to live forex or commodity prices, allowing for more adaptive and market-driven protocols.
2. NFT Pricing Models
Non-Fungible Token (NFT) marketplaces can integrate Pyth to price NFTs tied to real-world assets (e.g., tokenized art, real estate, or physical goods):
- Price feeds from Pyth can reflect the value of underlying assets, ensuring fair valuations.
- Fractionalized NFTs can adjust their token value based on real-time price changes of the underlying assets.
3. Cross-Chain Arbitrage
With Pyth’s cross-chain capabilities, DeFi traders can exploit arbitrage opportunities across multiple blockchains by:
- Fetching synchronized price feeds to identify discrepancies in asset prices across chains.
- Automating arbitrage bots that capitalize on price mismatches in real time.
4. Real-Time Risk Management
DeFi insurance platforms can use Pyth data to:
- Monitor asset prices and automatically adjust coverage or premium rates based on current market conditions.
- Trigger payouts or liquidation mechanisms promptly during significant market shifts.
Deploying the Contract
- Compile the smart contract using Solidity version
^0.8.0
. - Deploy the contract on a testnet like Sepolia or Goerli before the mainnet for the test.
- Use tools like Remix, Hardhat, or Truffle to verify and interact with your smart contract before integrating it with your plateforme
Conclusion
Pyth Network provides a robust, low-latency solution for fetching real-world price data in decentralized applications. By integrating Pyth into your smart contracts, you can build reliable and cost-effective systems for DeFi, trading platforms, and more. Additionally, you can enhance the security and performance of your smart contracts with expert guidance from our security company, SmartContract.tips.
In this guide, we covered:
- The importance of Pyth Network as an oracle solution.
- The step-by-step process to integrate Pyth into Solidity contracts.
- Common security risks and mitigation strategies.
- Best practices to ensure efficient and secure usage.
- Advanced use cases showcasing Pyth’s potential across DeFi and NFT ecosystems.
For developers building on Ethereum or other supported chains, Pyth simplifies access to institutional-grade financial data while minimizing costs.
For further reading and advanced use cases, visit the Pyth Documentation and explore how to take your smart contracts to the next level.