On-Chain Decision-Making: Implementing DAO Governance with Governor Contracts
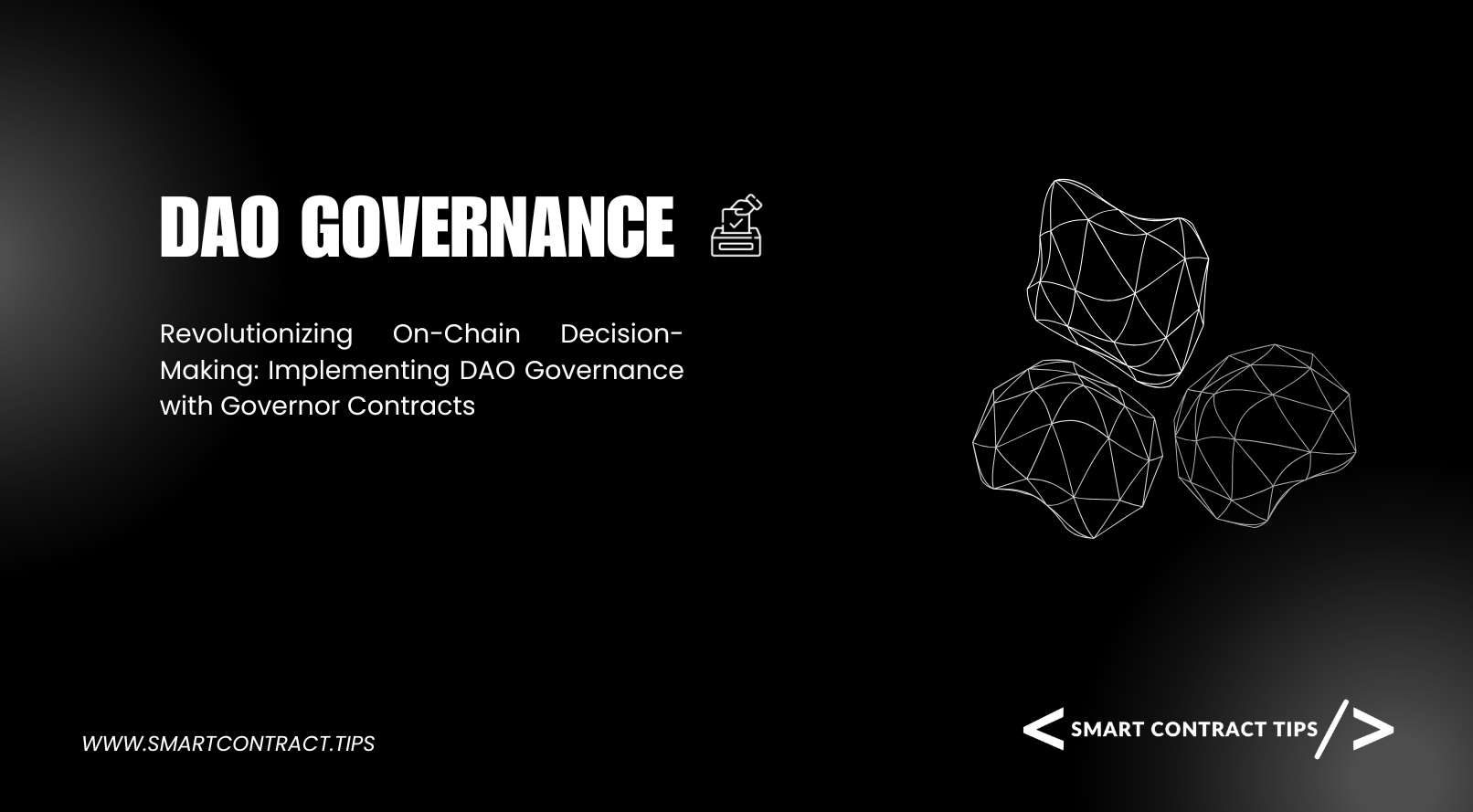
Introduction
Decentralized Autonomous Organizations (DAOs) have redefined the way we think about governance and decision-making in blockchain ecosystems. By enabling community-driven control over protocols, DAOs empower users to propose, vote, and implement changes without centralized oversight.
At the heart of most DAO implementations is the Governor Contract, a smart contract that handles governance proposals and voting. With frameworks like OpenZeppelin’s Governor contract, developers can easily set up robust DAO governance systems tailored to their project’s needs.
In this article, we will:
- Discuss the core principles of DAO governance and why it matters.
- Provide a step-by-step guide to implementing a Governor contract in Solidity.
- Explore customization options like voting mechanisms, proposal thresholds, and execution delays.
- Highlight potential risks and security measures in DAO governance.
- Present real-world use cases and code examples to demonstrate effective DAO implementation.
By the end of this guide, you’ll be equipped to design and deploy DAO governance systems that are transparent, secure, and adaptable to your blockchain project.
What Are DAOs and Governor Contracts?
Decentralized Autonomous Organizations
DAOs are community-governed organizations where decisions are made through token-weighted voting. Instead of relying on traditional hierarchies, DAOs operate on smart contracts that execute decisions automatically based on majority votes.
Governor Contracts
Governor contracts provide a framework for implementing governance in DAOs. They typically include functionalities for:
- Proposal Creation: Allowing members to suggest changes or actions.
- Voting: Enabling token holders to vote on proposals.
- Execution: Enacting approved proposals on-chain after a predefined delay.
Popular implementations, like OpenZeppelin’s Governor, make it easy to bootstrap governance while maintaining flexibility for customization.
Implementing DAO Governance Using Governor Contracts
Step 1: Import OpenZeppelin’s Governor Framework
OpenZeppelin provides a modular Governor framework that simplifies the implementation of DAO governance. Import the required modules:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/governance/Governor.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorCountingSimple.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorVotes.sol";
import "@openzeppelin/contracts/governance/extensions/GovernorTimelockControl.sol";
Step 2: Define the Governor Contract
Create a contract that inherits from OpenZeppelin’s Governor modules:
contract MyGovernor is Governor, GovernorCountingSimple, GovernorVotes, GovernorTimelockControl {
constructor(IVotes _token, TimelockController _timelock)
Governor("MyGovernor")
GovernorVotes(_token)
GovernorTimelockControl(_timelock)
{}
// Override required functions
function votingDelay() public pure override returns (uint256) {
return 1; // 1 block
}
function votingPeriod() public pure override returns (uint256) {
return 45818; // ~1 week in blocks (assuming 15s block time)
}
function quorum(uint256 blockNumber) public pure override returns (uint256) {
return 10000; // Minimum number of votes required for quorum
}
}
This example sets a voting delay of 1 block, a voting period of approximately one week, and a quorum requirement of 10,000 votes.
Step 3: Deploy the Contract
You need two additional components to deploy the Governor contract:
- Governance Token: A token that implements the
IVotes
interface (e.g., an ERC20Votes token). - Timelock Controller: A contract that enforces a delay before executing approved proposals.
Deploy the governance token, timelock controller, and then the Governor contract, passing the addresses of the first two as parameters.
Customizing Your Governor Contract
- Voting Mechanisms
Modify voting styles by extending different Governor modules:- GovernorCountingSimple: Simple yes/no/abstain voting.
- GovernorCountingQuadratic: Weighted voting based on quadratic voting principles.
- Proposal Thresholds
Set a minimum number of tokens required to create a proposal:function proposalThreshold() public pure override returns (uint256) { return 1000; // 1000 tokens required }
- Execution Delays
UseGovernorTimelockControl
to enforce a delay before executing proposals. This gives the community time to react to malicious proposals.
Here’s the updated section about calculating vote weight depending on the project’s tokenomics, such as staking or holding:
Customizing Vote Weight Based on Tokenomics
In many blockchain projects, voting power is not distributed equally. Instead, it is often calculated based on specific project mechanisms like token staking or holding duration. This ensures that governance decisions reflect the commitment and participation of token holders.
Vote Weight Calculation Strategies
- Holding-Based Weight
Assign more voting power to addresses based on the number of tokens held:- Linear Weight: Voting power is directly proportional to the token balance.
- Scaled Weight: Apply a multiplier to reward larger holders disproportionately.
Implementation Example:
function getVoteWeight(address voter) public view returns (uint256) { return token.balanceOf(voter); }
-
Staking-Based Weight
Encourage active participation by granting voting power only to staked tokens. This aligns governance with long-term project interests.Implementation Example:
function getVoteWeight(address voter) public view returns (uint256) { return stakingContract.getStakedAmount(voter); }
Here,
stakingContract
represents the contract managing token staking, andgetStakedAmount
retrieves the amount staked by the voter. - Time-Based Holding Weight
Reward long-term holders by increasing voting power based on the duration tokens are held:- Calculate the weight using a time factor (e.g., tokens held for over a year get a 2x multiplier).
- Encourage retention and reduce sell pressure.
Implementation Example:
mapping(address => uint256) public holdingStart; function setHoldingStart(address voter) external { if (holdingStart[voter] == 0) { holdingStart[voter] = block.timestamp; } } function getVoteWeight(address voter) public view returns (uint256) { uint256 balance = token.balanceOf(voter); uint256 holdingDuration = block.timestamp - holdingStart[voter]; uint256 multiplier = holdingDuration > 365 days ? 2 : 1; return balance * multiplier; }
- Hybrid Weight
Combine multiple strategies to create a hybrid model, such as:- 50% weight from staked tokens.
- 30% weight from held tokens.
- 20% weight from the holding duration.
Implementation Example:
function getVoteWeight(address voter) public view returns (uint256) { uint256 stakedWeight = stakingContract.getStakedAmount(voter) * 50 / 100; uint256 heldWeight = token.balanceOf(voter) * 30 / 100; uint256 holdingDuration = block.timestamp - holdingStart[voter]; uint256 durationMultiplier = holdingDuration > 365 days ? 2 : 1; uint256 durationWeight = (token.balanceOf(voter) * durationMultiplier) * 20 / 100; return stakedWeight + heldWeight + durationWeight; }
Integrating Custom Vote Weight
Modify your Governor contract to account for the custom vote weight in governance decisions. Override the _getVotes
function in OpenZeppelin’s Governor contract to include your logic:
contract MyCustomGovernor is Governor, GovernorVotes {
constructor(IVotes _token) Governor("MyCustomGovernor") GovernorVotes(_token) {}
// Override to calculate vote weight based on custom logic
function _getVotes(
address account,
uint256 blockNumber,
bytes memory params
) internal view override returns (uint256) {
uint256 stakedWeight = stakingContract.getStakedAmount(account) * 50 / 100;
uint256 heldWeight = token.balanceOf(account) * 30 / 100;
uint256 holdingDuration = block.timestamp - holdingStart[account];
uint256 durationMultiplier = holdingDuration > 365 days ? 2 : 1;
uint256 durationWeight = (token.balanceOf(account) * durationMultiplier) * 20 / 100;
return stakedWeight + heldWeight + durationWeight;
}
}
Security Risks and Mitigation
-
Sybil Attacks
Attackers could create multiple accounts to gain voting power.
Mitigation: Use quadratic voting or require staking tokens to deter bad actors. -
Malicious Proposals
Proposals with harmful intentions could pass if not detected in time.
Mitigation: Implement a review process and allow veto powers for trusted entities. -
Timelock Vulnerabilities
If the timelock controller is compromised, attackers could bypass delays.
Mitigation: Use multi-signature wallets to control timelock functions.
Real-World Use Cases
-
Protocol Upgrades
Enable token holders to vote on upgrading smart contracts without downtime. -
Treasury Management
Allow the community to decide how funds are allocated for project development. -
Fee Adjustments
Vote on transaction fees or staking rewards dynamically based on market conditions.
Conclusion
DAOs and Governor contracts are foundational to decentralized governance in blockchain ecosystems. By leveraging frameworks like OpenZeppelin’s Governor, developers can easily implement robust and secure DAO systems.
In this article, we explored:
- The role of DAOs and Governor contracts.
- How to implement and customize a Governor contract in Solidity.
- Security considerations and real-world applications.
DAO governance not only empowers communities but also ensures transparency and accountability in decentralized systems. As blockchain adoption grows, implementing effective governance will remain a cornerstone of innovation.